The Coding From Scratch Bootcamp Bundle
4 Courses & 57 Hours
Deal Price$24.99
Suggested Price$800.00
You save 96%
What's Included
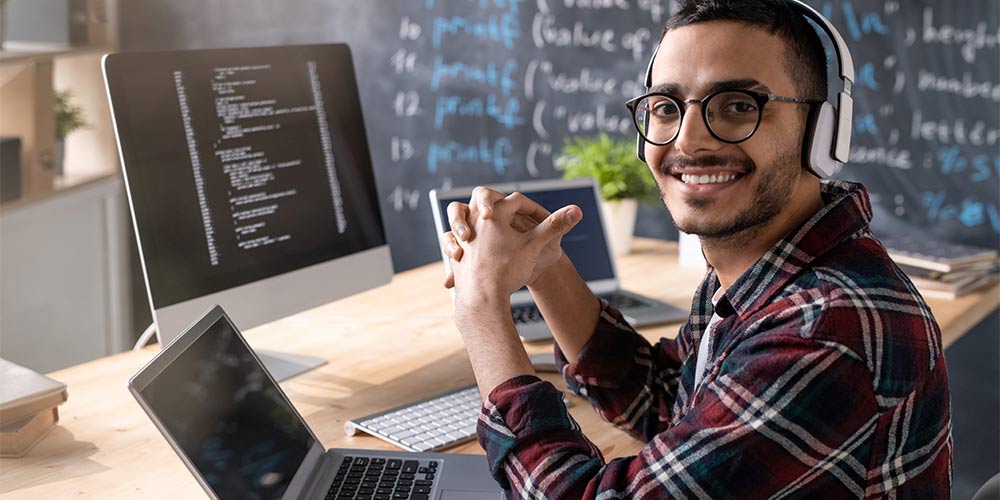
$200.00 Value
The Complete Python Masterclass 2022: Learn Python From Scratch
Ashutosh Pawar
343 Lessons (34h)
Lifetime
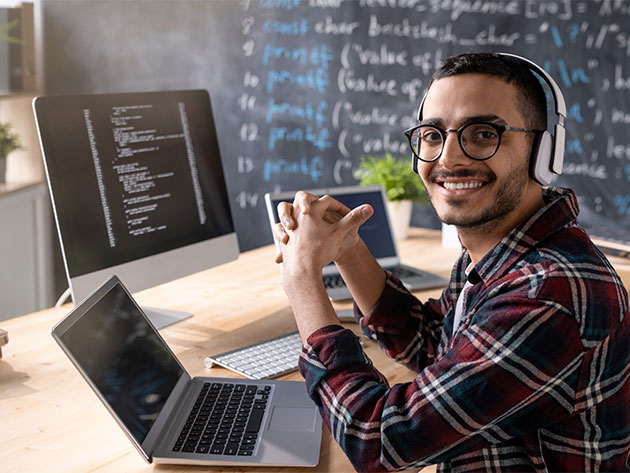
- Experience level required: All levels
- Access 343 lectures & 34 hours of content 24/7
- Length of time users can access this course: Lifetime
Course Curriculum
343 Lessons (34h)
- Your First Program
- Python basicsInstalling Python & PyCharm6:04Hello World Program In Python8:06Some Other Mathematical Operations6:14Strings In python5:00Accepting Input From User In Python5:06Performing Operations On String5:25Variables In Python6:33In Place Operators In Python6:28Writing Our Very First Python Program In PyCharm4:27Coding Challenge7:31Notes & Summary
- Control structuresIf Statement In Python8:32Elif Statement In Python5:37Lists In Pyton6:17List Operations8:32List Functions7:20Range Function5:10Range Function5:10For Loop8:35Boolean Logic5:57While Loop5:48Coding ChallengeCoding Challenge SolutionCoding ChallengeCoding Challenge SolutionNotes & Summary
- Functions & ModulesCode Reuse & Functions7:38Passing Arguments To Function6:28Making Function Return A Value4:37Passing Function As Argument5:19Modules In Python4:28Coding ChallengeCoding Challenge SolutionNotes & Summary
- Exception & File handlingErrors & Exceptions7:59Exception Handling4:54Finally Block3:23File Handling6:43Reading From File6:04Writing Data To Files4:16Appending To File2:01Coding ChallengeCoding Challenge SolutionCoding ChallengeCoding Challenge SolutionNotes & Summary
- Some More Types In PythonDictionaries7:10Dictionary Functions5:38Tuples3:41List Slicing5:19List Comprehension5:06String Formatting5:35String Functions7:57Numeric Functions2:45Coding ChallengeCoding Challenge SolutionCoding ChallengeCoding Challenge SolutionNotes & Summary
- Functional Programming In PythonFunctional Programming6:34Lambdas In Python5:06Map In Python6:06Filters In Python4:00Generators6:28Coding ChallengeCoding Challenge SolutionCoding ChallengeCoding Challenge SolutionCoding ChallengeCoding Challenge SolutionNotes & Summary
- Object Oriented Programming In PythonIntroduction To Classes & Objects5:18Methods3:01Creating Class & Class Attributes6:54Instance Attributes, Constructors & Self11:11Methods7:16OOP Way Of Writing Code8:35Inheritance6:47Multiple Inheritance4:07Multilevel Inheritance3:21Recursion In Python7:07Sets7:56Itertools9:23Operator Overloading In Python7:42Data Hiding In Python6:29Coding ChallengeCoding Challenge SolutionCoding ChallengeCoding Challenge SolutionNotes & Summary
- Regular Expressions In PythonIntroduction To Regular Expressions6:33Search & Find All5:03Find & Replace4:59The Dot Metacharacter3:33Caret & Dollar Metacharacter5:17Character Class6:03Star Metacharacter4:40Groups3:24
- Create GUI Apps Using TkinterTkinter: Hello World7:09Tkinter: Using Frames5:43Tkinter: Grid Layout6:32Tkinter: Self Adjusting Widgets5:00Tkinter: Handling Button Clicks4:03Tkinter: Using Classes10:39Tkinter: Creating Dropdowns11:41Tkinter: Toolbar8:03Tkinter: Statusbar3:25Tkinter: Message Box4:36Tkinter: Drawing5:21
- Project 1: Building Calculator Using TkinterBuilding A Calculator App Part 112:12Building A Calculator App Part 26:27Building A Calculator App Part 34:57Building A Calculator App Part 410:08
- Project 2: Building Database Apps With PostgreSQL & PythonWhat Are Databases & Why Do We Need Them5:57Introduction To PostgreSQL2:35Installing PostgreSQL On Windows3:32Installing PostgreSQL On Mac4:25Creating A Database6:38Creating A Database Table5:31Reading From Database2:49Creating Development Environment On Mac8:00Installing Psycopg2 On Mac2:27Establishing Connection5:32Creating Table4:45Inserting Data4:16Adding User Data To Database4:47Building A Tkinter & PostgreSQL App Part 111:00Building A Tkinter & PostgreSQL App Part 37:43Building A Tkinter & PostgreSQL App Part 25:13Building A Tkinter & PostgreSQL App Part 413:03Building A Tkinter & PostgreSQL App Part 56:05
- File Compression & Encoding In PythonPerforming Compression13:24Performing Decompression4:49Compression & Decompression Functions9:34Designing User Interface For Compression App12:54Compression Using GUI6:13Opening Files From Explorer6:20
- Text To Speech Converter Using PythonText-To-Speech App Part 15:53Text-To-Speech App Part 25:55Text-To-Speech App Part 37:29
- Implementing Password Hashing & Building A Password ValidatorIntroduction To Hashing9:52Implementing Hashing In Python9:40Building User Interface For Password Validation9:13
- Building A QR Code Generator With PythonQR code Generator Part 18:54QR code Generator Part 28:48
- Building A Video Downloader AppVideo Downloader App Part 18:32Video Downloader App Part 23:52Video Downloader App Part 33:12Video Downloader App Part 48:20Video Downloader App Part 53:44Video Downloader App Part 63:32
- Credit Card Validation Using Lhun's AlgorithmLhun's Algorithm Part 18:36Lhun's Algorithm Part 212:06Lhun's Algorithm Part 39:48
- Data Analysis With PythonIntroduction To Data Analysis7:05Installing Anaconda5:26Introduction To Series8:03Converting Dictionaries To Series3:31Introduction To Dataframe6:28Changing Column Sequence6:53Transposing A Dataframe5:35Reindexing Series & Dataframes7:50Deleting Rows & Columns4:12Arithmetic Operations8:43new python data analysis part_107:23Dataframe Arithmatic5:42Sorting According To Values3:11Handling Duplicate Values2:54Calculating Sum3:29Dropping NaN Values5:33Loading Data From Files4:57Creating Numpy Array3:21Another Way To Create An Array4:11Logspace & Linspace4:01Slicing Numpy Array7:01Advanced Indexing9:16Broadcasting5:26Analyzing File Data7:41Iterating Using NdIter3:23Matplotlib6:26
- Project 3: Sales Data Analysis Using Python & PandasReading From CSV File3:19Changing Themes3:39Indexing & Slicing A DataFrame9:39Selecting Rows On A Condition8:07Query Method6:36SUM, MAX, MIN7:08Groupby6:20Calculating Location Share11:04data analysis part 9 6:53Analyzing Members & Ratings6:13Writing Multiple Queries16:25Analyzing Daily Sales8:40Analyzing Sales By Month3:46Analyzing Sales By Hour4:10Analyzing Payment Types VS Hours3:46
- Building Web Applications With Django 3Introduction To Django6:30Installing Django On Windows2:52Installing Django On Mac2:12Creating Django Project On Windows7:04Creating Django Project On Mac3:50Understanding Significance Of Files6:45Running Django Project On Windows5:30Running Django Project On Mac4:32Creating A View8:36Creating Another View4:12What is a Database6:00Creating A Model4:10Making Migrations3:00Adding Objects To Database11:10Admin Panel8:40Passing Objects4:09Templates7:06Passing Context6:42Detail View Part 19:18Detail View Part 25:08Detail View Part 36:06Removing Hardcoded URLs4:53URLs For Myapp5:30Namespacing URLs5:29Adding Static Files9:08Adding Bootstrap8:02Using Base Template10:38Adding Image Field11:08Retrieving Images3:31Adding Own Images3:12Adding HTML Form8:53Submitting Data To Database10:04Edit Part 19:56Fixing Issues & Edit10:46Update Button2:40Delete Functionality11:07Design Touch up Index9:48Design Touch up Detail3:45
- Building A Todo Web App With Django 3How To Go From Idea To App9:49Installing Virtualenv7:24Setting Up The Project3:15Creating Model6:22Form View & Template6:53Handling Post Rrequest5:45Adding Bootstrap10:05Read Functionality8:29Two Functionalities On Same Page4:37Styling Part 19:23Styling Part 22:16Styling Part 36:15Delete Functionality9:43Add Functionality9:34Edit Funcctionality12:15Introduction To Class Based Views3:53ListView8:12DetailView6:35UpdateView9:28DeleteView4:04
- Project 5: Build REST API's With Python & DjangoIntroduction To REST API6:00Creating A REST API20:35Creating Endpoints For API8:37Adding Image Field10:34Filtering An API7:40Search Functionality4:10Adding Authentication15:33
- Project 6: Learn How To Crawl Websites Using PythonWeb Crawler Part 16:23Web Crawler Part 25:39Web Crawler Part 34:56Web Crawler Part 55:13Web Crawler Part 67:25Web Crawler Part 73:50Web Crawler Part 87:54Web Crawler Part 95:18Web Crawler Part 105:25Web Crawler Part 114:22Web Crawler Part 124:21Web Crawler Part 137:03Web Crawler Part 146:27Web Crawler Part 155:11Web Crawler Part 165:34Web Crawler Part 175:52Web Crawler Part 186:23Web Crawler Part 196:12Web Crawler Source Code
- Automation Using Selenium Web Driver & PythonIntroduction To Selenium2:00Installing Selenium3:46Opening A URL3:21Automating Google Search7:03Find Element By XPATH4:12Clicking Links5:19Refreshing A Webpage2:27Using Forward & Backward Buttons3:07Scroll & Get Current URL4:45Building A Facebook Auto Poster Bot13:23
- Network Programming In Python Using SocketsIP Address14:04Ports & Sockets12:29Creating The Client & Server14:09Sending A Message7:52Buffer10:22Messaging App7:58Sending Messages To Client10:01Send Message Functionality9:45Finishing The App8:58
- Image Processing With OpenCV & PythonIntroduction To OpenCV2:52Installing OpenCV2:41Reading & Displaying Images11:12Getting Video Form Webcam7:34Drawing On Images12:38Mouse Callback Events9:00Manipulating Pixels10:43Color Space4:59Object Tracking In Images14:07Tracking Objects In A video5:50Image Thresholding7:05Simple Thresholding6:20Adaptive Thresholding4:16Adaptive Thresholding Code6:14Geometric Transformation Of Images8:39Scaling & Transformation11:50Image Blurring5:48Image Blurring Code5:08Averaging3:39Gaussian Filtering3:41
- Regular Expressions RevisitedRegular Expressions Part 16:52Regular Expressions Part 18:27Introduction To Metacharacters3:37Star Metacharacter2:20Plus Metacharacter1:52Plus Metacharacter2:16Curly Braces1:48Curly Braces Example3:23Wildcard Introduction1:36Wildcard Example3:33Optional Metacharacter2:31Caret Metacharacter3:38Character Classes Part 14:59Character Classes Part 25:00
- REST APIs With Python & Django Rest FrameworkIntroduction To API10:39Installing Django Rest Framework4:07Creating Django App7:47Setting Up The API10:42Creating A Serializer7:53Adding Another Endpoint4:49Update & Delete Functionality4:38Adding Images To API11:17Making The API Consumable5:53Creating React App12:00Using API In React App7:45
The Complete Python Masterclass 2022: Learn Python From Scratch
AP
Ashutosh PawarAshutosh Pawar | Entrepreneur, Python, Java & Android Geek
4.5/5 Instructor Rating:
★ ★ ★ ★
★ ★
Ashutosh Pawar is the director of Optimum Solutions, a software firm based in Pune India which works in providing software solutions to small and medium enterprises across the globe and has catered to over 200+ clients globally. He holds a bachelor's degree in Information Technology (Computer Science) Engineering and has over 8+ years of developing scalable software infrastructure across multiple domains which include real estate, medicine & finance. Along with being a businessperson, he always had a passion for teaching and his goal is to pass on his knowledge to his students and empower them to achieve their goals in the IT sector.
Description
In this hands-on course, you will learn Python right starting from scratch to the level where you can build almost anything with it, be it a fully functional database-oriented web application or an automation tool. This course will teach you Python right from scratch from a very basic level and will gradually move you towards more advanced topics. It will not just cover all the Python basics but also the most popular Python libraries such as Django, Flask, Tkinter & Selenium.
4.5/5 average rating:
★ ★ ★ ★
★ ★
- Access 343 lectures & 34 hours of content 24/7
- Learn the latest version of Python (i.e Python 3)
- Learn the latest Django version (i.e Django 3)
- Understand & learn each and every Python concept
- Automate social media posts using Selenium
- Make Web-Applications using Python
- Make GUI based Applications in Python using Tkinter
- Make full-stack web apps using Python and Django 3
- Process images & videos using OpenCV
- Make your own web-scraping tool using Python
- Build database oriented desktop apps with Python, PostgreSQL & Tkinter
Specs
Important Details
- Length of time users can access this course: lifetime
- Access options: desktop & mobile
- Redemption deadline: redeem your code within 30 days of purchase
- Experience level required: all levels
- Have questions on how digital purchases work? Learn more here
Requirements
- Any device with basic specifications
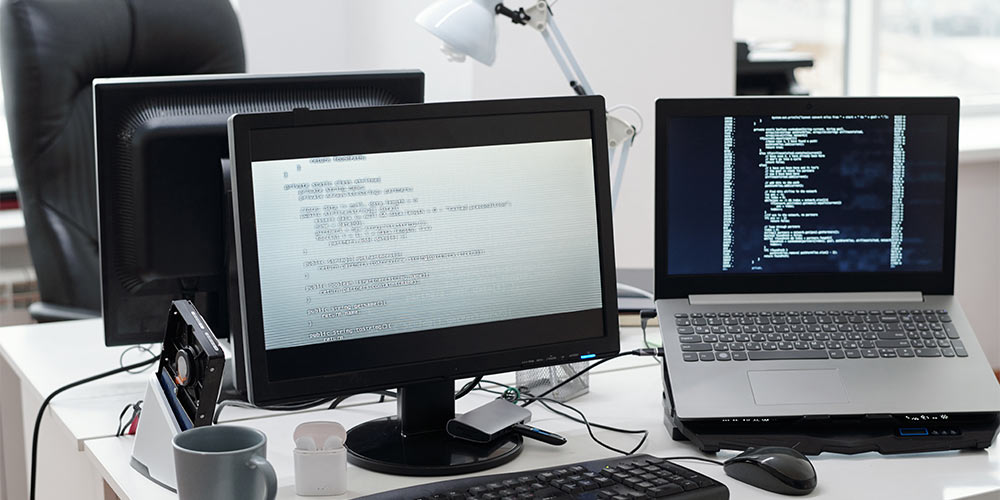
$200.00 Value
The Complete Java Masterclass: Learn Java From Scratch
Ashutosh Pawar
123 Lessons (14h)
Lifetime
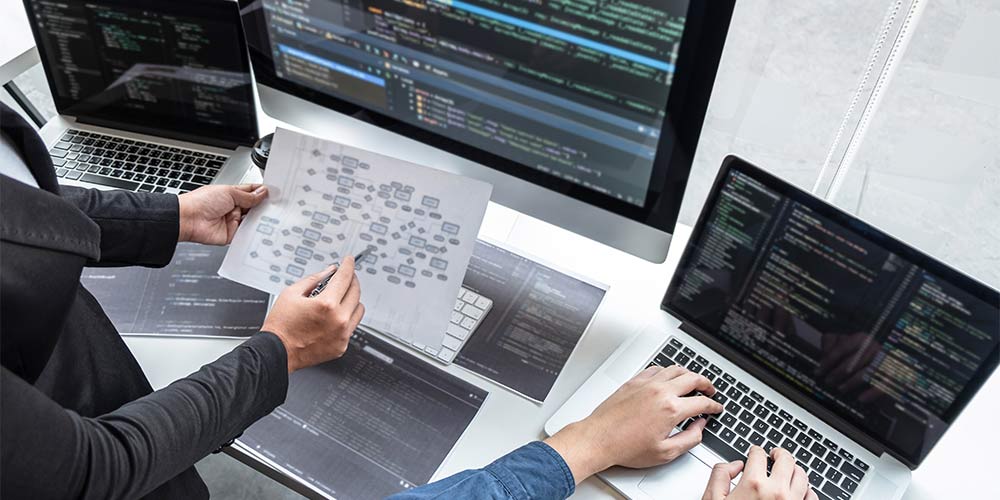
$200.00 Value
Tailwind CSS: A Modern Way to Build Websites Using CSS
Ashutosh Pawar
68 Lessons (6h)
Lifetime
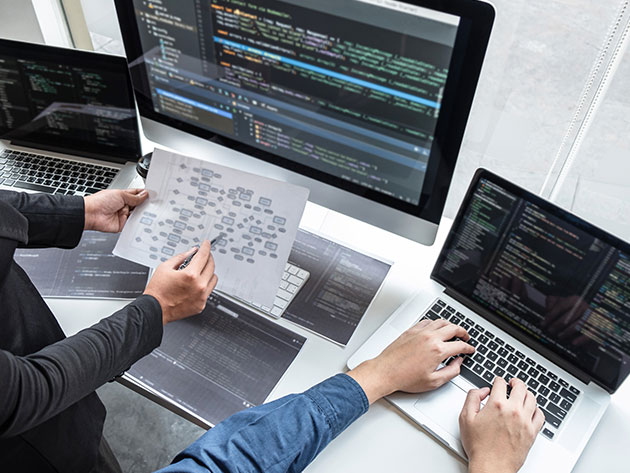
$200.00 Value
Sass Course for Beginners: Learn Sass & SCSS from Scratch
Ashutosh Pawar
37 Lessons (3h)
Lifetime
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.
1 Review
5/ 5
All reviews are from verified purchasers collected after purchase.
BW
Bernard W. Viney Jr.
Verified Buyer
The coding from scratch boot camp bundle has a wealth of useful information, especially for a beginner. I look forward to absorbing all of the coding knowledge I learn from this course.
Jun 6, 2022
Your Cart
Your cart is empty. Continue Shopping!
Processing order...