The Complete C Suite Programming Bundle
10 Courses & 80 Hours
Deal Price$49.99
Suggested Price$1,990.00
You save 97%
What's Included
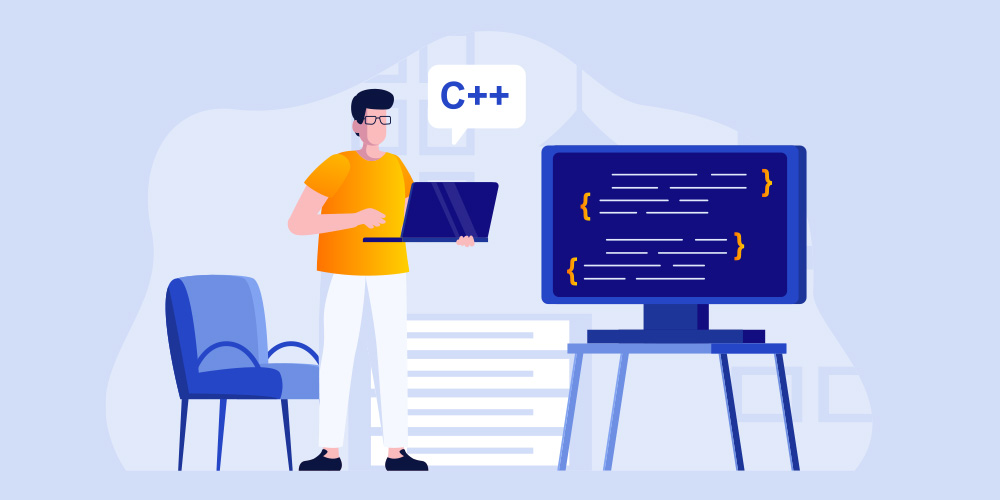
$199.00 Value
Complete Modern C++
Umar Lone
208 Lessons (18h)
Lifetime
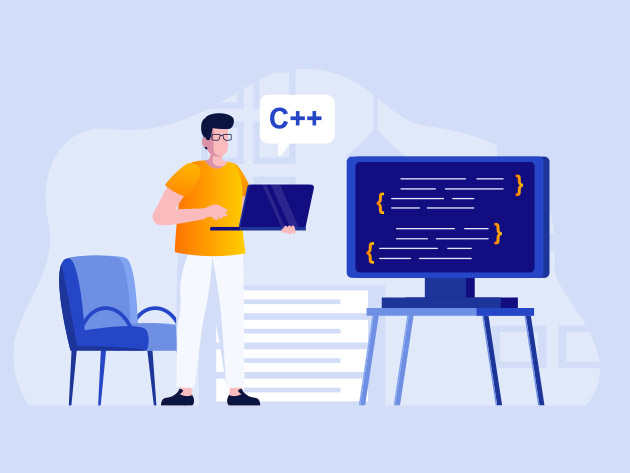
- Certification included
- Experience level required: Beginner
- Access 208 lectures & 18 hours of content 24/7
- Length of time users can access this course: Lifetime
Course Curriculum
208 Lessons (18h)
- Your First Program
- Software InstallationInstructor & Course Introduction3:01Source Code & Slide DeckRequired SoftwareVisual Studio 2015 Installation4:32Visual Studio 2017/19 Installation2:44CodeBlocks Installation5:06Cevelop Installation5:31XCode Installation3:32
- Fundamental Language FacilitiesWhat is C++?3:31First C++ Program9:12Compilation & Build3:09C++ Fundamental Types7:57Basic Console Input/Output5:56Functions - I9:13Functions - II9:05Debugging Basics5:40Uniform Initialization9:21Pointer Type8:25Reference Type5:31Reference Vs Pointer6:58const Qualifier4:19const Qualifier & Compound Types7:30Automatic Type Inference (auto)7:00Range-based For Loop - I4:32Range-based For Loop - II6:18Function Overloading9:07Default Function Arguments3:43Inline Functions7:36Function Pointers4:53Namespace9:24AssignmentsQuiz
- Memory Management - IDynamic Memory Allocation - I (malloc)9:36Dynamic Memory Allocation - II (new)6:19Dynamic Memory Allocation - III (new[])6:40Dynamic Memory Allocation - IV (2D Arrays)5:07
- Classes & ObjectsObject Oriented Programming Basics5:50Class5:30Constructor & Destructor4:28Structures2:38Non-static data member initialization4:39this Pointer4:46Constant Member Functions3:14Static Members5:12Copy Constructor - I4:52Copy Constructor - II4:40Delegating Constructor4:01Default & Deleted Functions5:18Quiz
- Move SemanticsValue Categories7:10Move Semantics Basics3:23Move Semantics Implementation3:44Rule of 5 & 014:30Copy Elision8:22std::move Function7:52Quiz
- Operator OverloadingOperator Overloading - I (Basics)8:28Operator Overloading - II - (Assignment Operator)4:17Operator Overloading - III (Global Operators)5:48Operator Overloading - IV - (Friend Functions)2:29Operator Overloading - V (Smart Pointers)5:04Operator Overloading - VI (Smart Pointers)4:34Operator Overloading - VII (Rules)1:55Type Conversion - I5:56Type Conversion - II (Primitive to User-defined)5:39Type Conversion - III (User-defined to primitive)2:50Initialization vs assignment5:18Quiz
- Memory Management - IIunique_ptr - I5:11unique_ptr - II10:10shared_ptr10:27weak_ptr - I8:49weak_ptr - II6:26Circular Reference & weak_ptr10:26
- More C++ Featuresenum Type - I3:42enum Type - II5:32C Style Strings6:49C++ std::string11:41String Streams9:45Constant Expressions (constexpr)9:24User-defined Literals5:59Initializer List8:27Automatic Dynamic Array (vector)8:43Union Type - I4:51Union Type - II4:56AssignmentsQuiz
- Object Oriented Programming with C++Inheritance & Composition6:01Inheritance & Access Modifiers3:54Project - I (Introduction)2:06Project - II (Account & Savings classes)6:59Project - III (Inheriting Constructor)6:31Project - IV (virtual Keyword)8:53Project - V (Virtual Mechanism Internals)7:11Project - VI (Virtual Mechanism Walkthrough)6:42Project VII (override & final Specifiers)6:13Project VIII (Upcasting & Downcasting)5:45Project - IX (RTTI Overview)7:11Project - X (RTTI Operators)3:1713. Inheritance - Project XI6:03Abstract Class6:03Multiple Inheritance9:21Quiz
- Exception HandlingIntroduction to Exception Handling7:58Catch Handlers3:58Stack Unwinding6:31Nested Exceptions5:10Exceptions in Constructors/Destructors7:06noexcept Specifier8:20Quiz
- File I/ORaw String Literals4:03Experimental Filesystem Library3:49Overview of File I/O6:44Error Handling in Files5:43File Copy Utility3:29Character Input/Output & Seeking7:22Binary Input/Output8:30Assignments
- TemplatesIntroduction to Templates7:48Template Argument Deduction & Instantiation6:27Explicit Specialization5:54Nontype Template Arguments7:14Perfect Forwarding - I7:23Perfect Forwarding - II5:32Variadic Templates - I9:47Variadic Templates - II4:50Class Templates8:39Class Explicit Specialization - I5:41Class Explicit Specialization - II4:02Class Partial Specialization6:52Alias Templates8:07Static Assertion4:19Type Traits7:21Assignments
- Lambda ExpressionsFunction Objects - I6:39Function Objects - II6:57Lambda Expressions & Syntax4:36Lambda Expressions Internals5:00Lambda Capture List - I8:25Lambda Capture List - II4:07Lambda Capture List - III5:57Generalized Lambda Capture4:33AssignmentsQuiz
- Standard Template LibraryStandard Library Components2:50std::array4:02std::vector4:38std::deque2:21std::list & std::forward_list4:59std::set & std::multiset5:42std::map & std::multimap7:34Unordered Containers Overview4:38std::unordered set & std::unordered map5:48Custom Hasher2:42Containers Comparison5:04Algorithms - I8:15Algorithms - II7:04AssignmentQuiz
- ConcurrencyConcurrency Basics5:42Thread Creation5:47Passing Arguments To Threads4:18Thread Synchronization6:17std::lock_guard2:45More Thread functions7:48Task-based Concurrency - I5:07Task-based Concurrency - II3:44Task Launch Policies4:38std::future Functions5:07std::promise5:36Exception Propagation In Threads3:15
- C++17 Core Language AdditionsDeprecated & Removed Features3:53Changes To Existing Features3:12Attributes5:58Feature Test Macros5:50if/switch With Initialization5:47Inline Variables5:45Nested Namespaces1:51noexcept Specifier Changes3:35constexpr Lambda7:47Structured Binding8:07Argument Evaluation Order6:36Mandatory Copy Elision - I4:28Mandatory Copy Elision - II4:33
- C++17 Template AdditionsClass Template Argument Deduction5:41Fold Expressions - I4:05Fold Expressions - II4:26Fold Expressions - III5:51Fold Expressions - IV2:34Type Trait Suffixes3:22Compile-Time if - I7:42Compile-Time if - II4:42
- C++17 Standard Library Additionsstd::optional - I5:18std::optional - II3:50std::optional - III3:37std::variant - I5:57std::variant - II4:34std::variant - III (Visitation)5:58std::any6:33std::string_view - I8:39std::string_view - II8:13Filesystem Library - I (path)5:49Filesystem Library - II (directory_entry)7:00Filesystem Library - III (Directory Functions)5:09Filesystem Library - IV (File Permissions)5:44Parallel Algorithms - I3:49Parallel Algorithms - II7:32Parallel Algorithms - III4:24
Complete Modern C++
UL
Umar LoneUmar Lone | Trainer, Developer & Founder at Poash Technologies
4.5/5 Instructor Rating:
★ ★ ★ ★
★ ★
Umar is a civil engineer who found his calling in software development. He never worked as a Civil Engineer as he jumped at the first chance and started teaching C++ & Visual C++ 15 years ago. Currently, Umar trains software professionals in various software companies in different technologies, such as Modern C++, Advanced C++, STL, Design Patterns, Android, Unity, Linux, etc. He is very passionate about teaching and has trained more than 20,000 software professionals in a teaching career spanning more than 17 years. An avid gamer, Umar is currently trying his hand at game development in Unity & Unreal. He has a few Android applications to his credit, including one on Design Patterns.Description
This course teaches C++ as an object-oriented language with modern features. It focuses on teaching C++ concepts, both old and new, with clear examples. It builds upon the basic language facilities used to build more complex programs with classes, operator overloading, composition, inheritance, polymorphism, templates, concurrency, and others. It even digs deep into assembly to understand few concepts better. After every few topics, a quiz is presented that tests your understanding of the previous topics. Have fun learning Modern C++.
4.5/5 average rating:
★ ★ ★ ★
★ ★
- Access 208 lectures & 18 hours of content 24/7
- Learn C++ concepts, both old & new, with clear examples
- Use C++ as an object-oriented language
- Demystify function & class templates
- Use STL components in your applications
- Write real-world applications in C++
- Apply Modern C++ (C++11/14/17) in programs
Specs
Important Details
- Length of time users can access this course: lifetime
- Access options: desktop & mobile
- Certificate of completion included
- Redemption deadline: redeem your code within 30 days of purchase
- Updates included
- Experience level required: beginner
- Have questions on how digital purchases work? Learn more here
Requirements
- Basic programming knowledge in any computer language
- Modern C++ compiler, preferably Visual Studio 2015/17/19 community (or XCode, Clang, g++, Cevelop, Eclipse CDT, Code::Blocks)
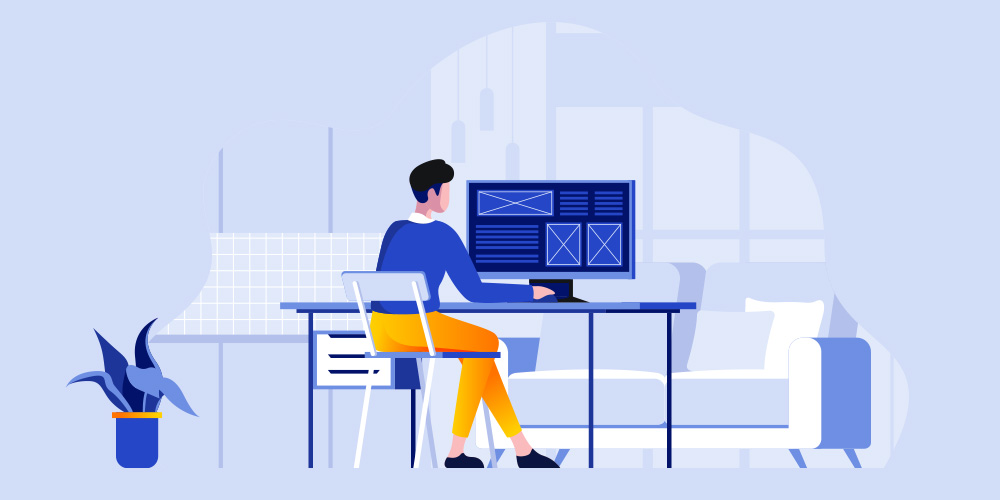
$199.00 Value
Master C# And SQL by Building Applications
Avetis Ghukasyan
55 Lessons (6h)
Lifetime
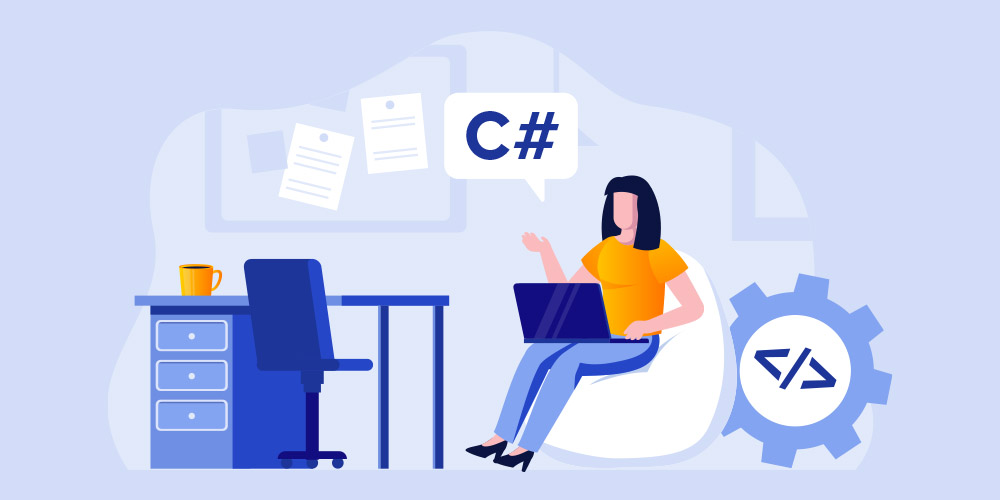
$199.00 Value
Learn C# by Building Applications
Avetis Ghukasyan
99 Lessons (13h)
Lifetime
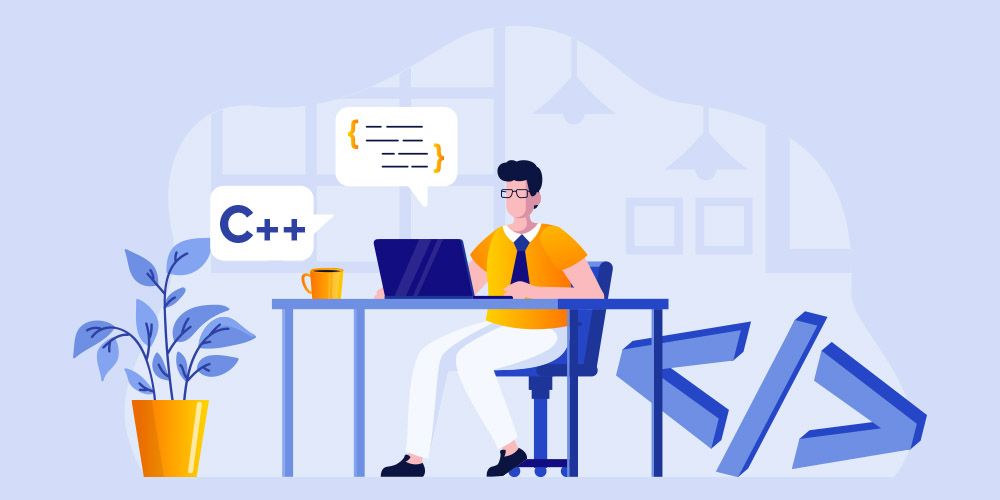
$199.00 Value
The Complete Introduction to C++ Programming
Yassin Marco
32 Lessons (4h)
Lifetime
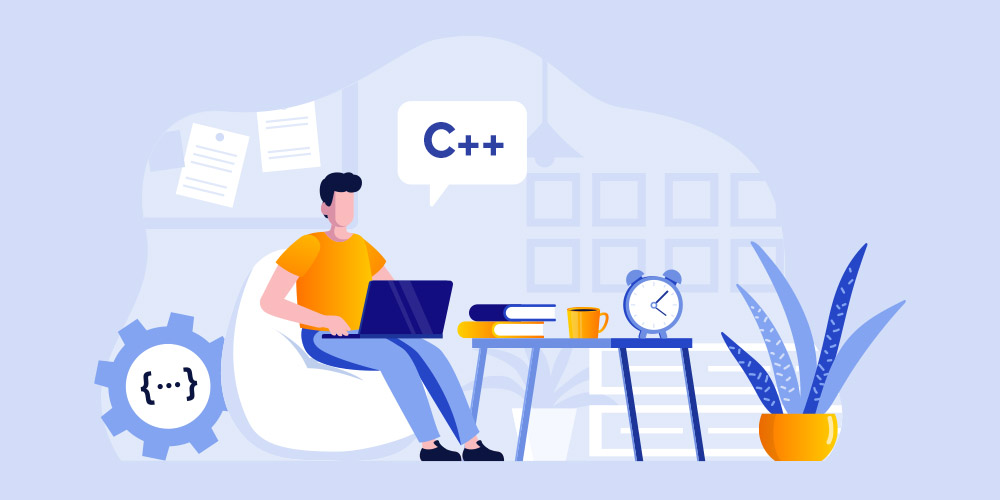
$199.00 Value
Structural Design Patterns in Modern C++
Umar Lone
92 Lessons (7h)
Lifetime
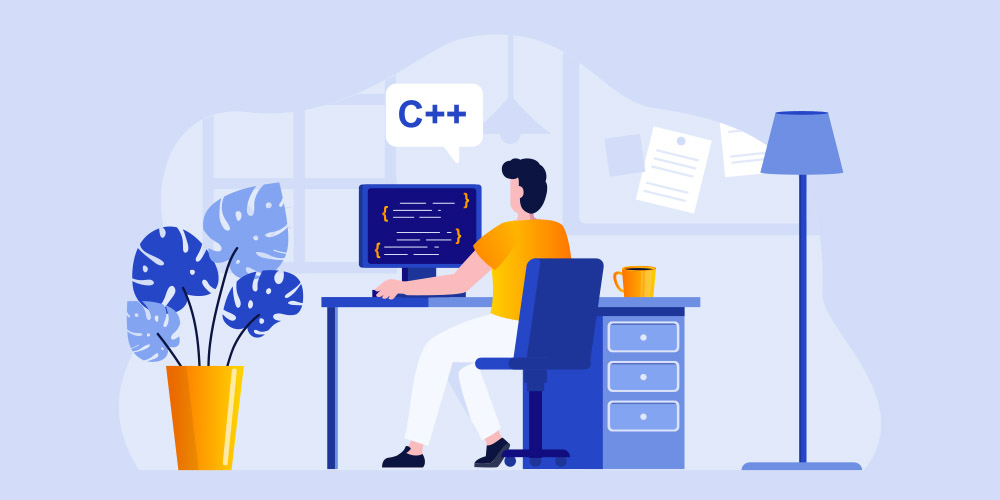
$199.00 Value
Creational Design Patterns in Modern C++
Umar Lone
88 Lessons (7h)
Lifetime
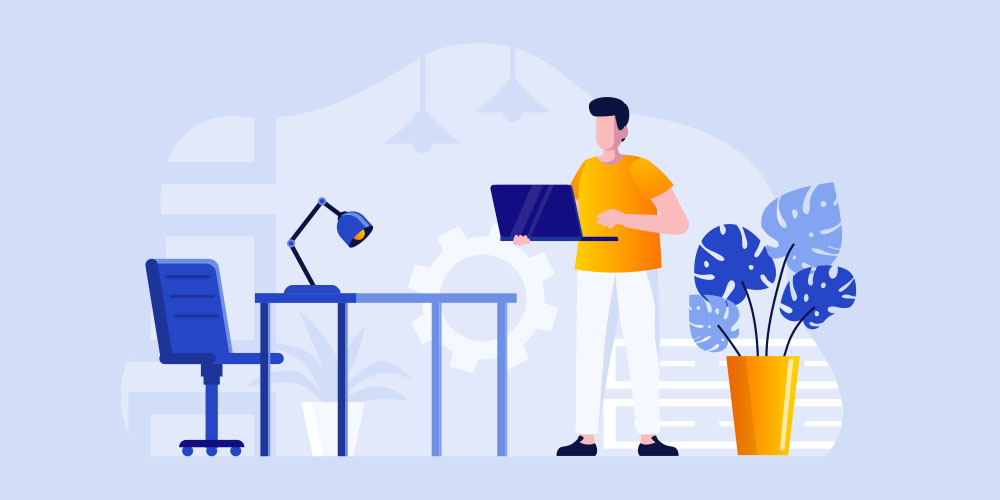
$199.00 Value
Object Oriented Analysis, Design & Programming with UML
Umar Lone
124 Lessons (10h)
Lifetime
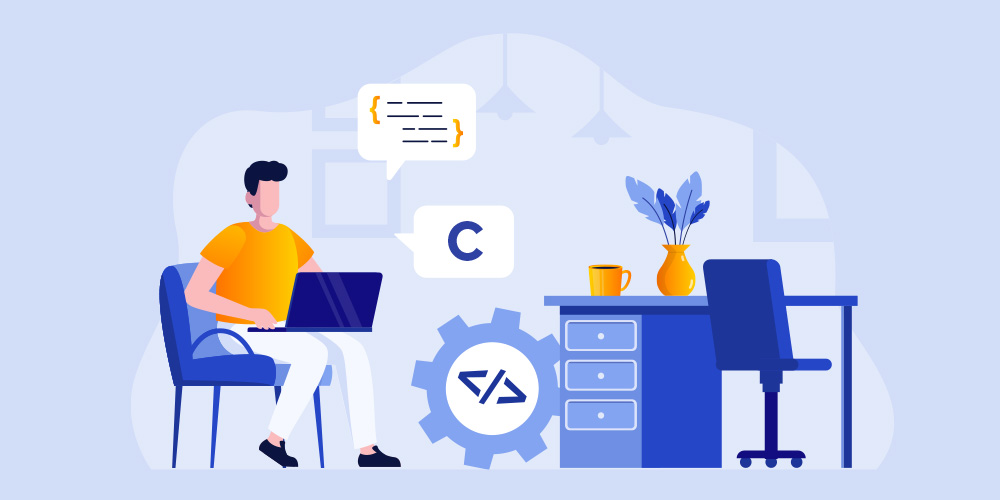
$199.00 Value
Detecting Memory Leaks in C/C++ Applications
Umar Lone
72 Lessons (6h)
Lifetime
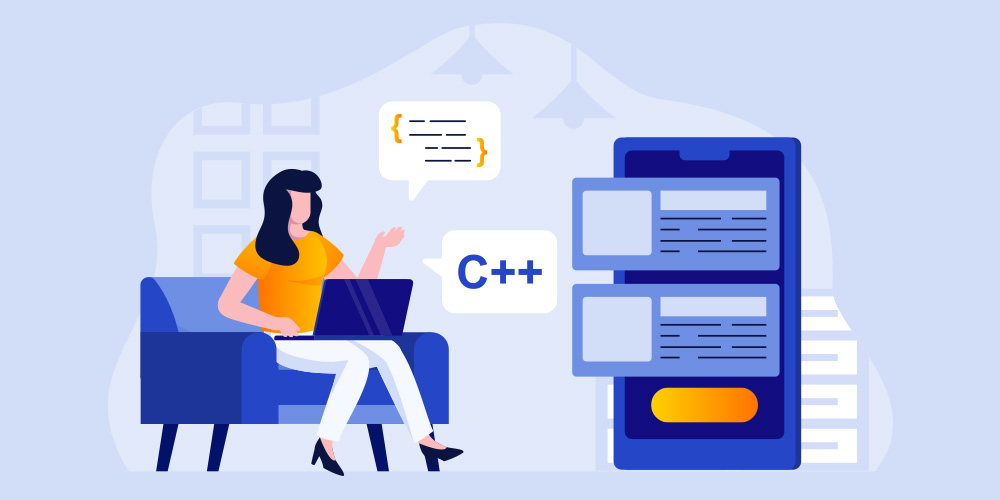
$199.00 Value
C/C++ Pointers & Applications
Umar Lone
72 Lessons (6h)
Lifetime
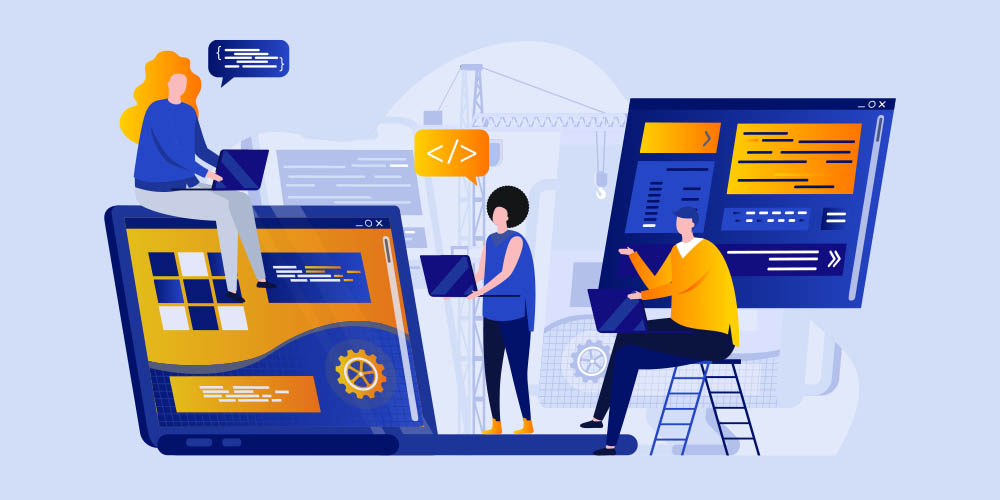
$199.00 Value
Beginner Object Oriented Programming In C# and .NET Core
Avetis Ghukasyan
26 Lessons (3h)
Lifetime
Terms
- Unredeemed licenses can be returned for store credit within 30 days of purchase. Once your license is redeemed, all sales are final.
Your Cart
Your cart is empty. Continue Shopping!
Processing order...